Already seen this part? Check out Part 1, Part 2, and Part3
In part 1, we covered the very basics. A one-line Get-ADUser query, a simple form with one button and output textbox.
In this entry, we’ll add more functionality that:
If you remember, we started off with this form:
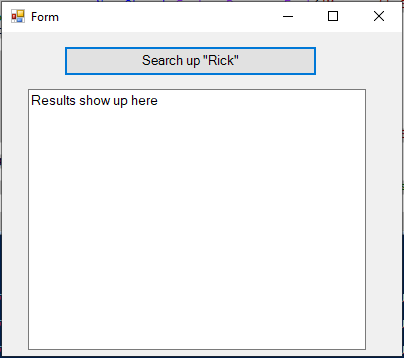
Simple winform with a button, a textbox, and a function.
Now, we’re going to take input from a user, and display the output to a datagridview instead of a textbox. A textbox is usually fine for output, but with a Get-ADUser query, there are multiple fields we usually want to look at. Text boxes don’t do text wrapping very well, and the output is kind of messy. Also in using a grid, it neatly sorts everything based on some criteria we create.
Here’s a sample of what the box looks like afterwards in PoshGUI:
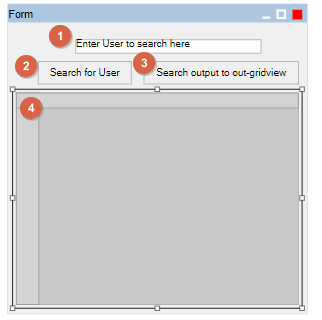
I’ve labelled each major component, and listing the names in the Powershell code for easier reference:
Textbox1
= This is the input from the end user. Or more accurately, we’ll be capturing the ‘text’, in the form of $textbox1.text into a variable, and using that in our Get-ADUser search parameter.Button1
= This executes the search query with the captured text from textbox1. The output from this will go into the 4th component: the datagridview.Button2
= This will execute the exact same search, but outputs it to an external gridviewer. I’ll go through some of the pro’s and con’s of this method later.Datagridview1
= output ofGet-Aduser
shows up here.
As I stated earlier, a textbox is usually fine for some simple output. A datagridview is handy since it puts all the results into columns that are resizable, sortable and we can copy directly from it. I listed two different ways to use grid form with Button 2 and the Datagridview1. I’ll cover more about what each does soon.
Below is the completed source code that:
- Makes use of the “search for User” button (AKA, ‘$button1’)
- Takes input from the textbox (AKA, ‘$Textbox2.text’)
- Uses a function ‘ADSearch’ which we defined the parameters
- Ouputs the result to a gridview embedded into the form.
I’ll go into more detail about the Gridviewer output, the variables, then we’ll create some code for error checking.
$results = $null #reset the $results variable each run
$server = “10.0.0.101”
$Credential = “contoso.com\administrator”
Add-Type -AssemblyName System.Windows.Forms
[System.Windows.Forms.Application]::EnableVisualStyles()
$Form = New-Object system.Windows.Forms.Form
$Form.ClientSize = New-Object System.Drawing.Point(400,389)
$Form.text = “Form”
$Form.TopMost = $false
$Button1 = New-Object system.Windows.Forms.Button
$Button1.text = “Search for User”
$Button1.width = 124
$Button1.height = 30
$Button1.location = New-Object System.Drawing.Point(41,52)
$Button1.Font = New-Object System.Drawing.Font(‘Microsoft Sans Serif’,10)
<#—–Calls a function ‘ADSearch’——-#>
$Button1.Add_MouseClick({ADSearch($Textbox2.text)})
$TextBox2 = New-Object system.Windows.Forms.TextBox
$TextBox2.multiline = $false
$TextBox2.text = “Enter User to search here”
$TextBox2.width = 247
$TextBox2.height = 20
$TextBox2.location = New-Object System.Drawing.Point(91,23)
$TextBox2.Font = New-Object System.Drawing.Font(‘Microsoft Sans Serif’,10)
$DataGridView1 = New-Object system.Windows.Forms.DataGridView
$DataGridView1.width = 376
$DataGridView1.height = 282
$DataGridView1.location = New-Object System.Drawing.Point(12,94)
$Button2 = New-Object system.Windows.Forms.Button
$Button2.text = “Search output to out-gridview”
$Button2.width = 206
$Button2.height = 30
$Button2.location = New-Object System.Drawing.Point(182,52)
$Button2.Font = New-Object System.Drawing.Font(‘Microsoft Sans Serif’,10)
$Form.controls.AddRange(@(
$TextBox2
$Button1,
$Button2,
$DataGridView1
))
[system.windows.forms.application]::run($form) #Runs form with runtimes – this is a trial and error thing
Function ADSearch {
<#——function ‘ADSearch’ runs Get-ADUser query, stores into $results variable——#>
$wc = ‘*’ #Wildcard character to assist with the text search
$Namesearch = $wc + $textbox2.text + $wc #appends the wildcard characters to the search, infront and behind, stores into the $NameSearch Variable
$results= get-Aduser -Filter {name -like $Namesearch} -Server $Server -Credential $Credential #This is the Query itself
<#—–Display output to the embedded DataGridView——-#>
$DataGridView1.DataSource = [System.Collections.ArrayList]([System.Object[]](,$results | Sort-Object SamAccountName, Name))
}
Let’s look at the variables:
$results = $null #reset the $results variable each run
$server = "10.0.0.101"
$Credential = "contoso.com\administrator"
This will come in handy later when we call the Get-ADUser query. By passing variables, it becomes a little easier to change the script to fit our needs. Especially if you’re dealing with credentials or domains.
Now let’s look at the datagridview:
$DataGridView1.DataSource = [System.Collections.ArrayList]([System.Object[]](,$results | Sort-Object SamAccountName, Name))
Breaking down each component:
$DataGridView1.DataSource
This is the datagriviewd itself. By default it’s already in a neatly arranged set of columns and rows. Makes sense when we use it for a large amount of data with multiple fields. Makes for easier parsing if we’re looking for something.
[System.Collections.ArrayList]([System.Object[]]
Since the Datagrid arranges the data, we need to store the information into an array. If we didn’t, it would all be string information. Some data does not translate into string information nicely (such as ‘account enabled’, which would just translate to a 1 or a 0).
(,$results
An array by default starts with a ‘0’ (zero) value. To display the results of the AD search, we stored it into the $results variable. By putting the comma in front “,” this tells the array to display all the contents from the beginning of the array.
| Sort-Object SamAccountName, Name))
The Pipe and Sort-Object commands sort the values accordingly by SamAccountName, then by Name. These values were imported by the original get-aduser command. You could actually list this by any value that get-Aduser is able to pull, such as ‘displayname’, ’email’, etc…
Read up on Part3 of this tutorial, where I’ll be adding an error checking ability, as well as using the other button for a different style of output.
[ivory-search 404 "The search form 3350 does not exist"]